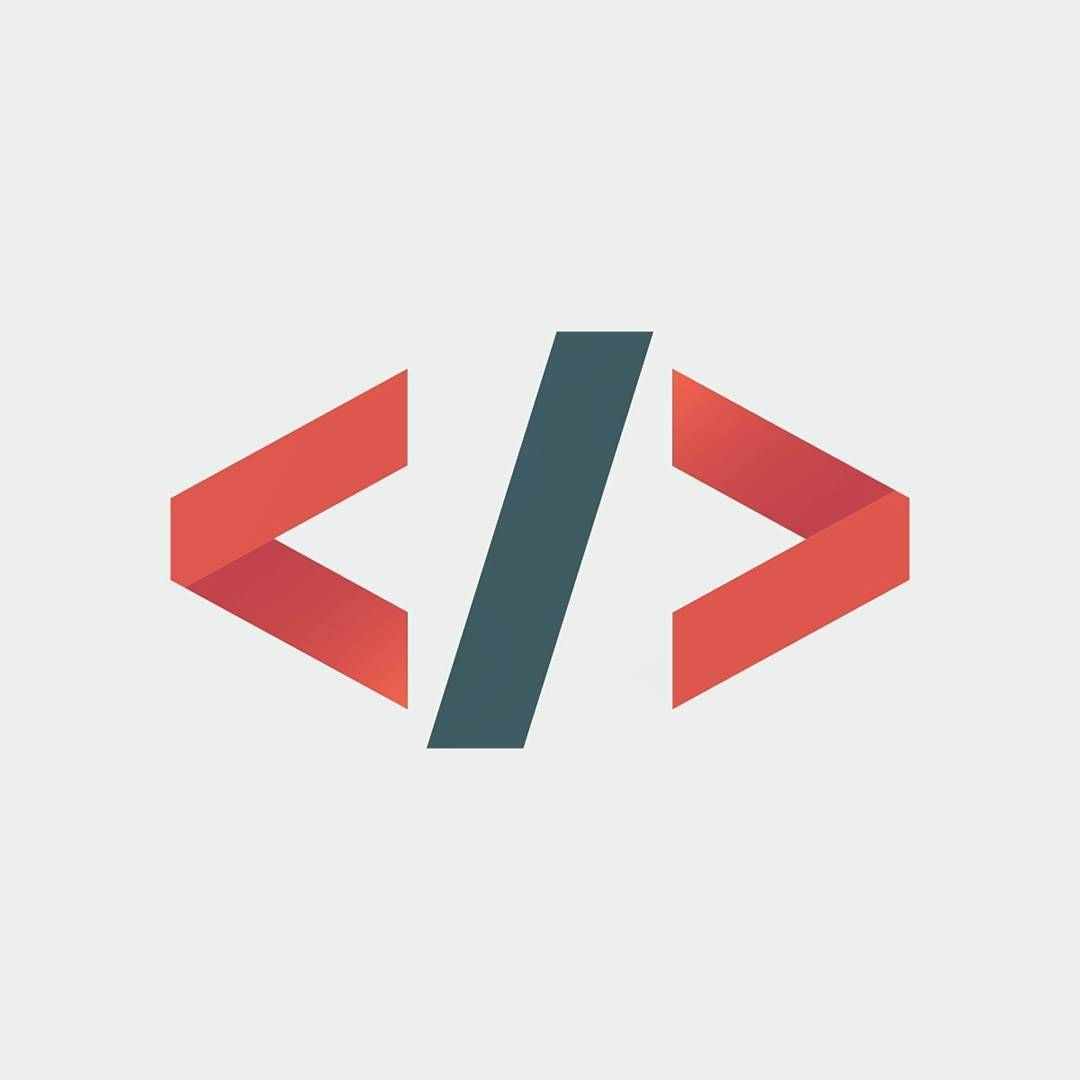
Variables
Every variable holds a value, which is the information associated with that variable.
For example:
message = "Hello world!"
print(message)
In this case the value is the text “Hello world!”
When it processes the first line, it associates the text “Hello Python world!” with the variable message. When it reaches the second line, it prints the value associated with message to the screen
You can change the value of a variable in your program at any time, and Python will always keep track of its current value.
Naming and Using Variables
When you’re using variables in Python, you need to adhere to a few rules and guidelines. Breaking some of these rules will cause errors.
variable rules:
1) Variable names can contain only letters, numbers, and underscores.
2) They can start with a letter or an underscore, but not with a number.
3) Spaces are not allowed in variable names
4) Avoid using Python keywords and function names as variable names.
✔️Some correct variable names: message, message_1, stu_data.
❌Some incorrect variable names: 1message, message@1, stu data.
Strings
The first data type we’ll look at is the string. Strings are quite simple at first glance, but you can use them in many different ways.
A string is simply a series of characters. Anything inside quotes is considered a string in Python, and you can use single or double quotes around your strings like this:
"This is a String"
'This is also a String'
Methods in String
1) len()
It will print the length of the given string (include every special case).
name = "Crack Python"
print(len(name))
This will display the following:
>>>12
2) title()
it displays each word in titlecase, where each word begins with a capital letter.
name = "crack python"
print(name.title())
This will display the following:
>>>Crack Python
In this example, the lowercase string is stored in the variable name. The method title() appears after the variable in the print() statement. A method is an action that Python can perform on a piece of data. The dot (.) after name in name.title() tells Python to make the title() method act on the variable name. Every method is followed by a set of parentheses, because methods often need additional information to do their work. That information is provided inside the parentheses.
3) upper() and lower()
upper()
It displays in uppercase, where each letter of word is capital.
lower()
It displays in lowercase, where each letter of word is smallcase.
name = "Crack Python"
print(name.upper())
print(name.lower())
This will display the following:
>>>CRACK PYTHON
crack python
4) capitalize()
It will display the given string with first letter capital and rest in lowercase. x
name = "crack Python"
print(name.capitalize())
This will display the following:
>>>Crack python
Above are some most common functions that are used in string. We will discuss more function with details in later part.
Combining or Concatenating Strings
first_name = "Crack"
last_name = "Python"
print(first_name + last_name)
This will display the following:
>>>CrackPython
Python uses the plus symbol (+) to combine strings. In this example, we use + to create a full name by combining a first_name, and a last_name.
By default, there will not be space between two strings when they are concatenated with "+" operator.
You can do like this:
first_name = "Crack"
last_name = "Python"
print(first_name + " " + last_name)
This will display the following:
>>>Crack Python
This method of combining strings is called concatenation. You can use concatenation to compose complete messages using the information you’ve stored in a variable.
Numbers
1) Integers
You can add (+), subtract (-), multiply (*), and divide (/) integers in Python.
>>> 2 + 3
5
>>> 3 - 2
1
>>> 2 * 3
6
>>> 3 / 2
1.5
In a terminal session, Python simply returns the result of the operation. Python uses two multiplication symbols to represent exponents:
>>> 3 ** 2
9
>>> 3 ** 3
27
>>> 10 ** 6
1000000
Python supports the order of operations too, so you can use multiple operations in one expression. You can also use parentheses to modify the order of operations so Python can evaluate your expression in the order you specify.
For example:
>>> 2 + 3*4
14
>>> (2 + 3) * 4
20
2) Floats
Python calls any number with a decimal point a float. This term is used in most programming languages, and it refers to the fact that a decimal point can appear at any position in a number.
Simply enter the numbers you want to use, and Python will most likely do what you expect:
>>> 0.1 + 0.1
0.2
>>> 0.2 + 0.2
0.4
>>> 2 * 0.1
0.2
>>> 2 * 0.2
0.4
But be aware that you can sometimes get an arbitrary number of decimal places in your answer:
>>> 0.2 + 0.1
0.30000000000000004
>>> 3 * 0.1
0.30000000000000004
This happens in all languages and is of little concern. Python tries to find a way to represent the result as precisely as possible, which is sometimes difficult given how computers have to represent numbers internally.
Avoiding Type Errors with the str() Function
Often, you’ll want to use a variable’s value within a message. For example, say you want to wish someone a happy birthday. You might write code like this:
age = 23
message = "Happy " + age + "rd Birthday!"
print(message)
You might expect this code to print the simple birthday greeting, Happy 23rd birthday! But if you run this code, you’ll see that it generates an error:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: can only concatenate str (not "int") to str
This is a type error. Python knows that the variable could represent either the numerical value 23 or the characters 2 and 3.
When you use integers within strings like this, you need to specify explicitly that you want Python to use the integer as a string of characters.
You can do this by wrapping the variable in the str() function, which tells Python to represent non-string values as strings.
age = 23
message = "Happy " + str(age) + "rd Birthday!"
print(message)
This will display the following:
Happy 23rd Birthday!
Comments
Comments are an extremely useful feature in most programming languages. Everything you’ve written in your programs so far is Python code. As your programs become longer and more complicated, you should add notes within your programs that describe your overall approach to the problem you’re solving.
A comment allows you to write notes in English within your programs.
In Python, the hash mark (#) indicates a comment. Anything following a hash mark in your code is ignored by the Python interpreter. For example:
# Say hello to everyone.
print("Hello Python people!")
Python ignores the first line and executes the second line.
Hello Python people!
Summary
In this chapter you learned to work with variables. You learned to use descriptive variable names and how to resolve name errors and syntax errors when they arise. You learned what strings are and how to display strings using lowercase, uppercase, and titlecase.
You started working with integers and floats, and you read about some unexpected behavior to watch out for when working with numerical data. You also learned to write explanatory comments to make your code easier for you and others to read.
Write a comment ...